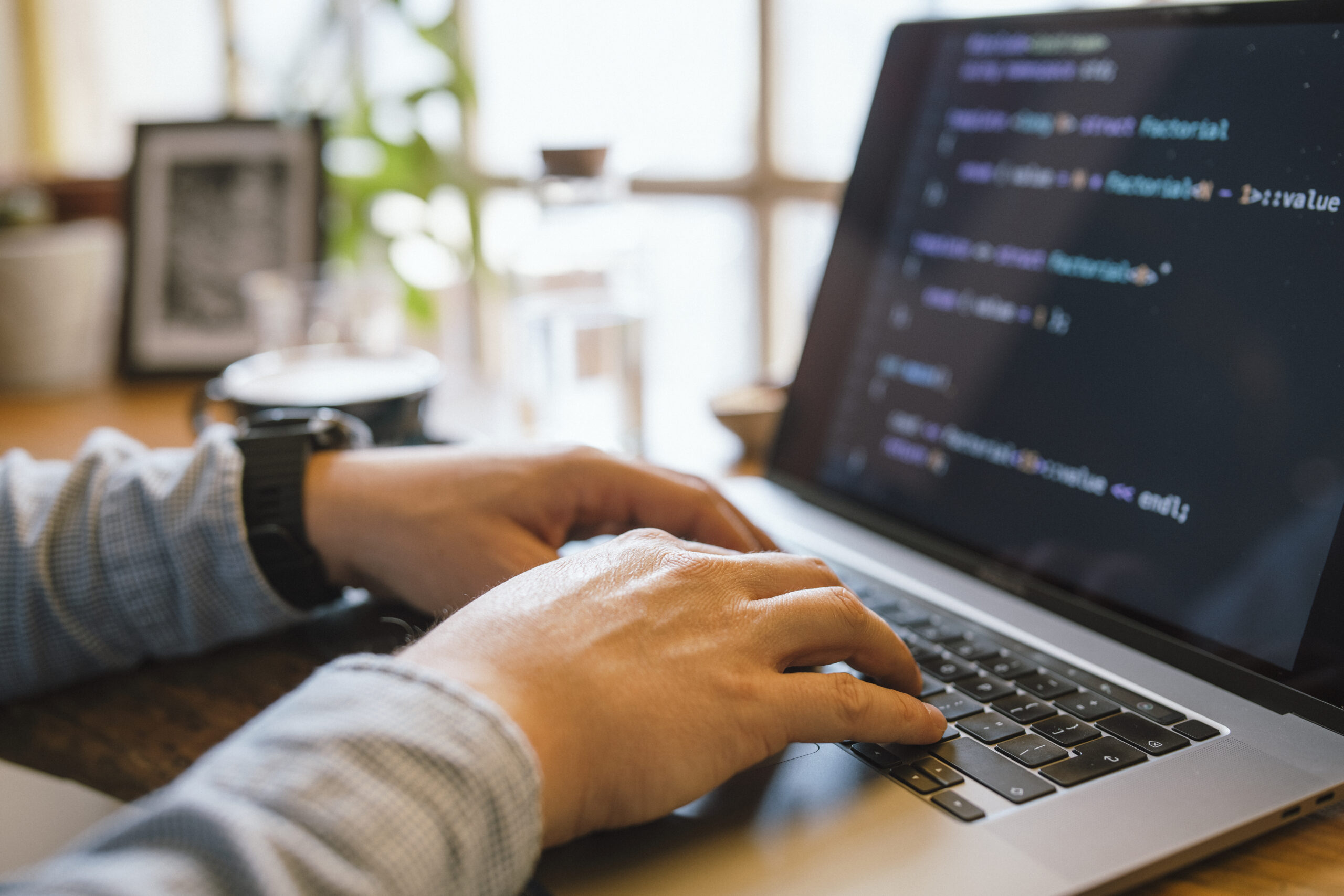
Debugging is one of the most important — but generally missed — capabilities within a developer’s toolkit. It is not pretty much repairing broken code; it’s about being familiar with how and why things go Incorrect, and Understanding to think methodically to solve issues proficiently. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging competencies can save hours of disappointment and considerably enhance your productiveness. Listed below are many approaches that can help builders degree up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Instruments
One of the fastest means developers can elevate their debugging abilities is by mastering the tools they use each day. Whilst composing code is just one Portion of growth, figuring out tips on how to communicate with it properly for the duration of execution is equally essential. Contemporary development environments come Outfitted with powerful debugging capabilities — but lots of builders only scratch the surface of what these applications can perform.
Consider, for example, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These equipment enable you to set breakpoints, inspect the worth of variables at runtime, step as a result of code line by line, and also modify code over the fly. When used correctly, they Enable you to notice just how your code behaves in the course of execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for front-close developers. They permit you to inspect the DOM, keep track of community requests, check out serious-time efficiency metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can switch annoying UI problems into workable responsibilities.
For backend or technique-amount developers, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control above functioning processes and memory administration. Studying these tools can have a steeper Understanding curve but pays off when debugging general performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become snug with version Handle devices like Git to understand code background, uncover the precise moment bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates likely further than default configurations and shortcuts — it’s about creating an intimate familiarity with your growth natural environment in order that when troubles occur, you’re not missing in the dead of night. The greater you are aware of your applications, the more time you'll be able to devote solving the actual issue as opposed to fumbling by way of the procedure.
Reproduce the condition
One of the most critical — and often missed — ways in helpful debugging is reproducing the issue. Prior to jumping into your code or making guesses, builders will need to make a dependable natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, correcting a bug gets a activity of prospect, normally bringing about wasted time and fragile code adjustments.
Step one in reproducing a difficulty is gathering just as much context as is possible. Request questions like: What steps resulted in the issue? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The more element you've got, the easier it becomes to isolate the precise circumstances less than which the bug happens.
As you’ve collected enough information, try and recreate the issue in your neighborhood atmosphere. This may imply inputting a similar facts, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, contemplate crafting automatic tests that replicate the edge conditions or condition transitions associated. These exams not simply assist expose the issue and also prevent regressions Later on.
From time to time, the issue could be natural environment-distinct — it'd occur only on specified functioning systems, browsers, or below distinct configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a phase — it’s a way of thinking. It requires patience, observation, plus a methodical tactic. But as you can regularly recreate the bug, you might be already halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment additional successfully, check prospective fixes securely, and talk a lot more Obviously using your crew or users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Go through and Recognize the Error Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. As an alternative to viewing them as aggravating interruptions, developers should master to take care of error messages as direct communications from your method. They often show you just what exactly took place, in which it happened, and in some cases even why it transpired — if you know the way to interpret them.
Commence by studying the information meticulously and in full. Quite a few builders, particularly when under time force, look at the initial line and instantly get started building assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines — read and fully grasp them initial.
Crack the error down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable patterns, and Mastering to recognize these can dramatically increase your debugging procedure.
Some glitches are imprecise or generic, and in those situations, it’s crucial to look at the context wherein the error transpired. Look at associated log entries, input values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger challenges and provide hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint troubles speedier, cut down debugging time, and become a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood while not having to pause execution or stage with the code line by line.
An excellent logging method begins with being aware of what to log and at what degree. Frequent logging amounts contain DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic data through growth, Details for standard gatherings (like prosperous start out-ups), Alert for probable troubles that don’t break the application, Mistake for actual complications, and Deadly once the method can’t go on.
Stay clear of flooding your logs with abnormal or irrelevant data. An excessive amount logging can obscure important messages and decelerate your program. Concentrate on key events, state changes, enter/output values, and significant selection details in your code.
Structure your log messages Evidently and persistently. Consist of context, for instance timestamps, request IDs, and performance names, so it’s easier to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Primarily useful in output environments the place stepping by code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a well-imagined-out logging solution, you'll be able to decrease the time it takes to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently establish and fix bugs, developers have to tactic the process just like a detective fixing a thriller. This mentality helps break down sophisticated troubles into workable sections and stick to clues logically to uncover the basis bring about.
Get started by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality issues. Just like a detective surveys a crime scene, gather as much related details as it is possible to with no leaping to conclusions. Use logs, take a look at cases, and user reports to piece together a transparent photograph of what’s going on.
Upcoming, sort hypotheses. Check with on your own: What could be causing this actions? Have any variations a short while ago been designed to your codebase? Has this challenge transpired just before below very similar conditions? The aim is always to narrow down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Endeavor to recreate the trouble inside a controlled atmosphere. If you suspect a certain operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code inquiries and Allow the outcome lead you nearer to the truth.
Pay shut consideration to little aspects. Bugs typically conceal inside the least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and client, resisting the urge to patch The difficulty without having fully knowledge it. Short-term fixes may perhaps conceal the actual problem, only for it to resurface afterwards.
Finally, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for future troubles and assistance Other people fully grasp your reasoning.
By thinking similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated programs.
Write Assessments
Crafting tests is one of the most effective strategies to transform your debugging skills and General advancement effectiveness. Assessments not simply aid catch bugs early but in addition function a security Web that offers you confidence when creating adjustments in your codebase. A properly-examined software is much easier to debug mainly because it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with unit tests, which focus on individual functions or modules. These little, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a examination fails, you quickly know where by to glance, appreciably minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following previously becoming preset.
Upcoming, integrate integration exams and end-to-close assessments into your workflow. These aid make sure that many portions of your application work together effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If something breaks, your assessments can tell you which Component of the pipeline unsuccessful and below what ailments.
Creating checks also forces you to Believe critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge scenarios. This degree of knowledge By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. As soon as the check fails continually, you can center on fixing the bug and observe your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In brief, producing checks turns debugging from a irritating guessing match right into a structured and predictable process—assisting you catch additional bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tough problem, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging applications is solely stepping absent. Using breaks aids you reset your brain, minimize stress, and sometimes see The problem from a new viewpoint.
When you are also close to the code for also extended, cognitive fatigue sets in. You may start out overlooking evident problems or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less efficient at trouble-resolving. A brief stroll, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down in front of a monitor, mentally caught, is Gustavo Woltmann coding not only unproductive and also draining. Stepping away allows you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It may well really feel counterintuitive, In particular below limited deadlines, however it essentially leads to a lot quicker and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak spot—it’s a smart method. It presents your brain Area to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to increase to be a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries once the bug is settled: What triggered it? Why did it go unnoticed? Could it are caught previously with superior techniques like device screening, code opinions, or logging? The solutions generally expose blind spots with your workflow or comprehension and allow you to Create more robust coding patterns going ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring issues or popular faults—which you could proactively keep away from.
In staff environments, sharing Whatever you've realized from a bug with all your friends may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same problem boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from frustration to curiosity. In lieu of dreading bugs, you’ll commence appreciating them as essential portions of your improvement journey. In fact, a number of the best developers are not the ones who generate excellent code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer on account of it.
Summary
Improving your debugging skills will take time, exercise, and patience — nevertheless the payoff is big. It makes you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.